Weeks 13-20 "Tripple trouble!"
Match 3 games are fun, addictive, and simple to code (Apparently). With time restraints being a constant reminder and fear, I decided against going with my original idea and instead work on something a little simpler. It would also ease concerns with my limited coding knowlege.
Unlike the others, I didn't start on paper, I instead went straight into digital work. Creating simple looking character heads that would be used in the game, with inspiration coming from Pokemon Link where Pokemon faces were matched instead of something like Candy Crush that used objects.
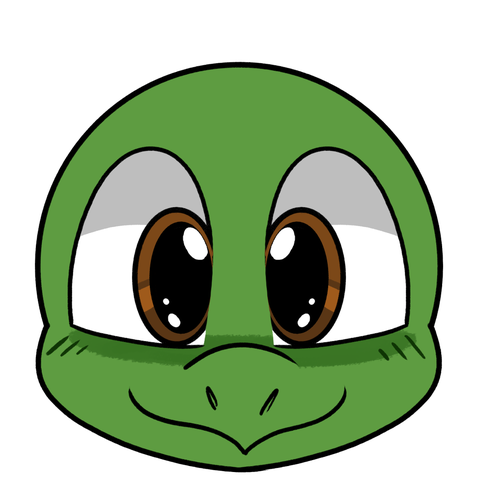
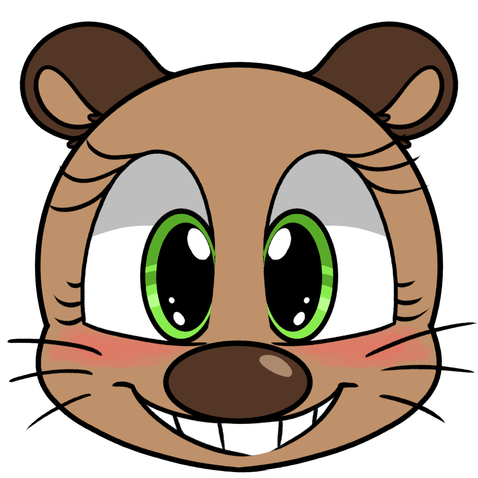
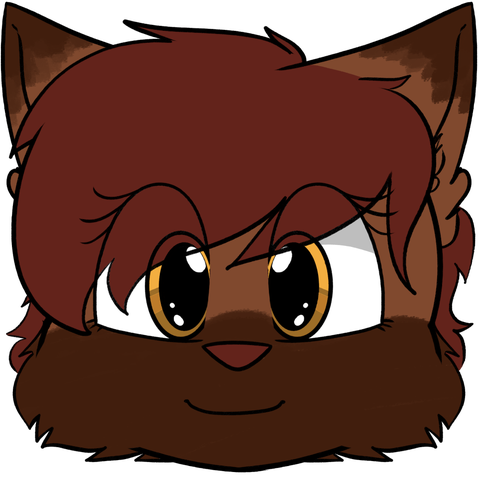
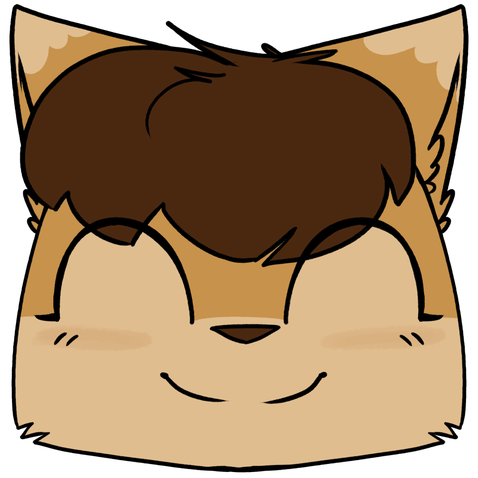
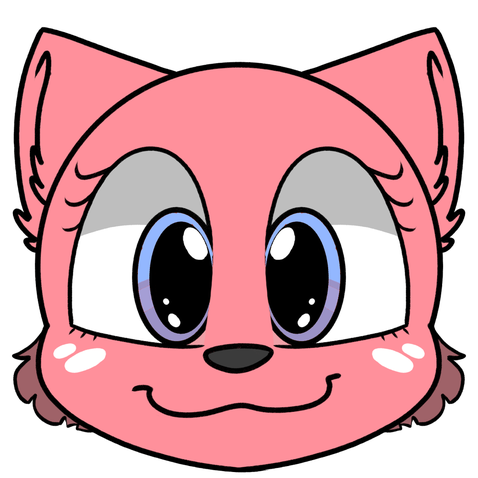
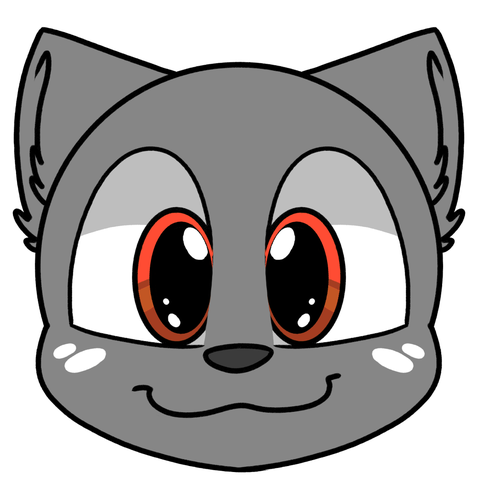
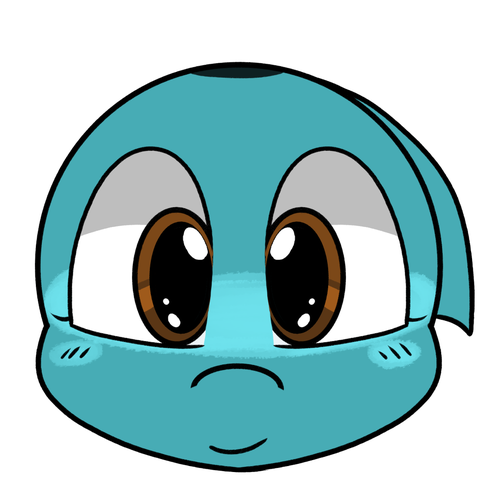
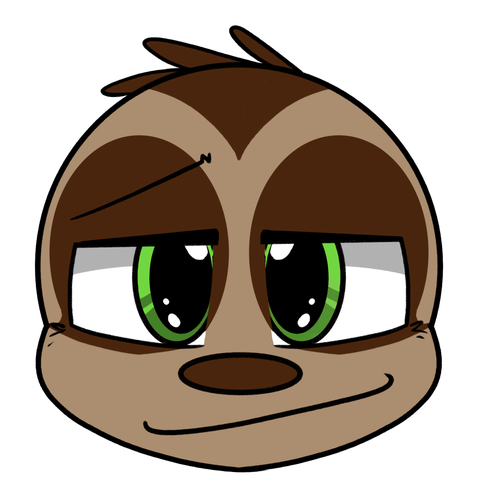
The tutorial I was following called for 8 objects, and thus these characters were chosen and drawn to all fit within the grid without any clipping. Some art from the tutorial was used to make it playable. Buttons amd menu layout was kept as it honestly looked the same as how I would have done it anyway. Tests on mobile proved worthwhile and the game had 3 playable levels.
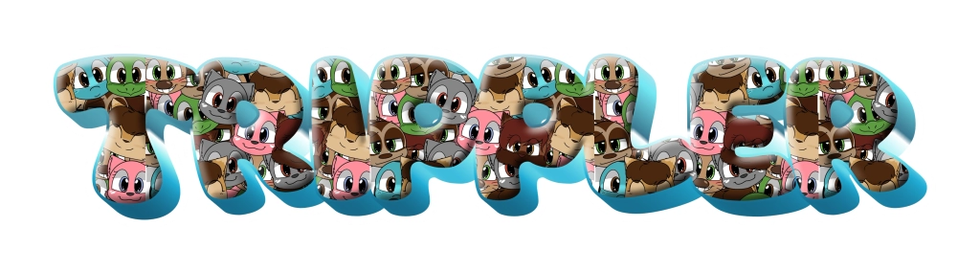
Decided on a name and design for the title. "Trippler" sounds fun to say, hints at what the gameplay is and is memorable for children. And since I really like this game, I even whipped up some further material, possibly for advertising or for use in the game, like an icon that represents the game on another screen... Whatever the future would bring.
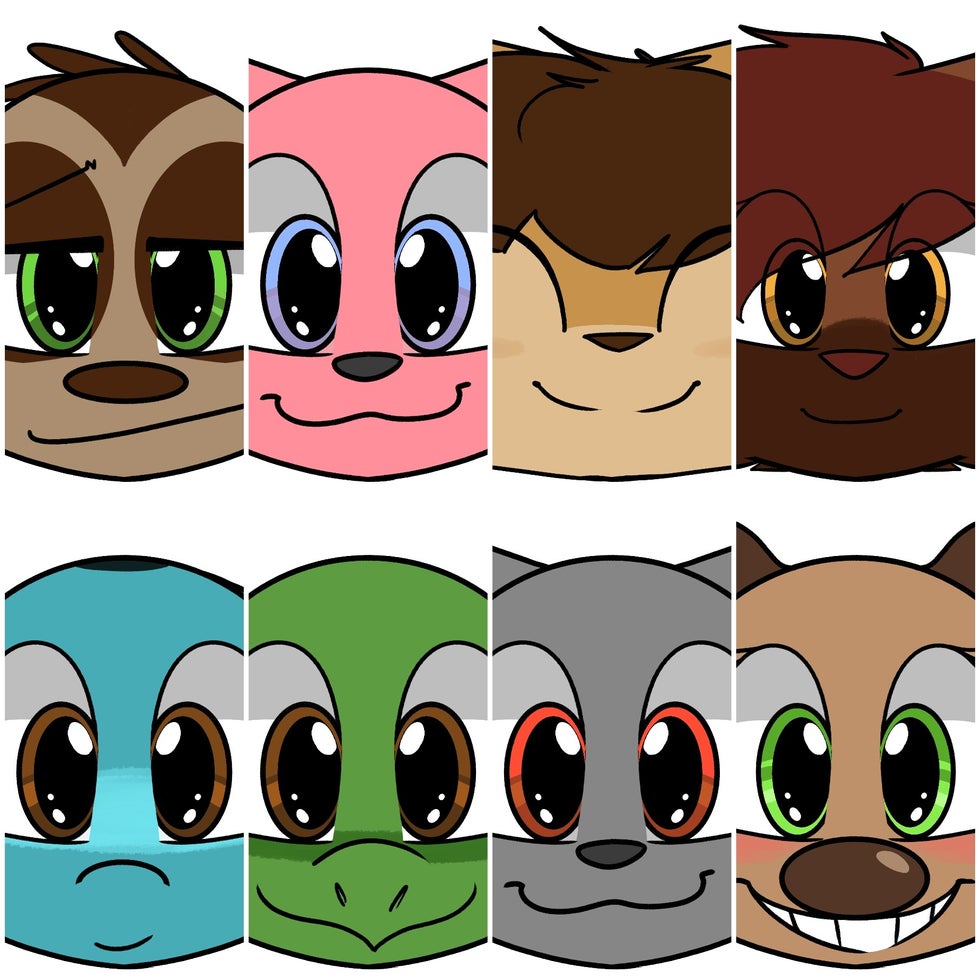
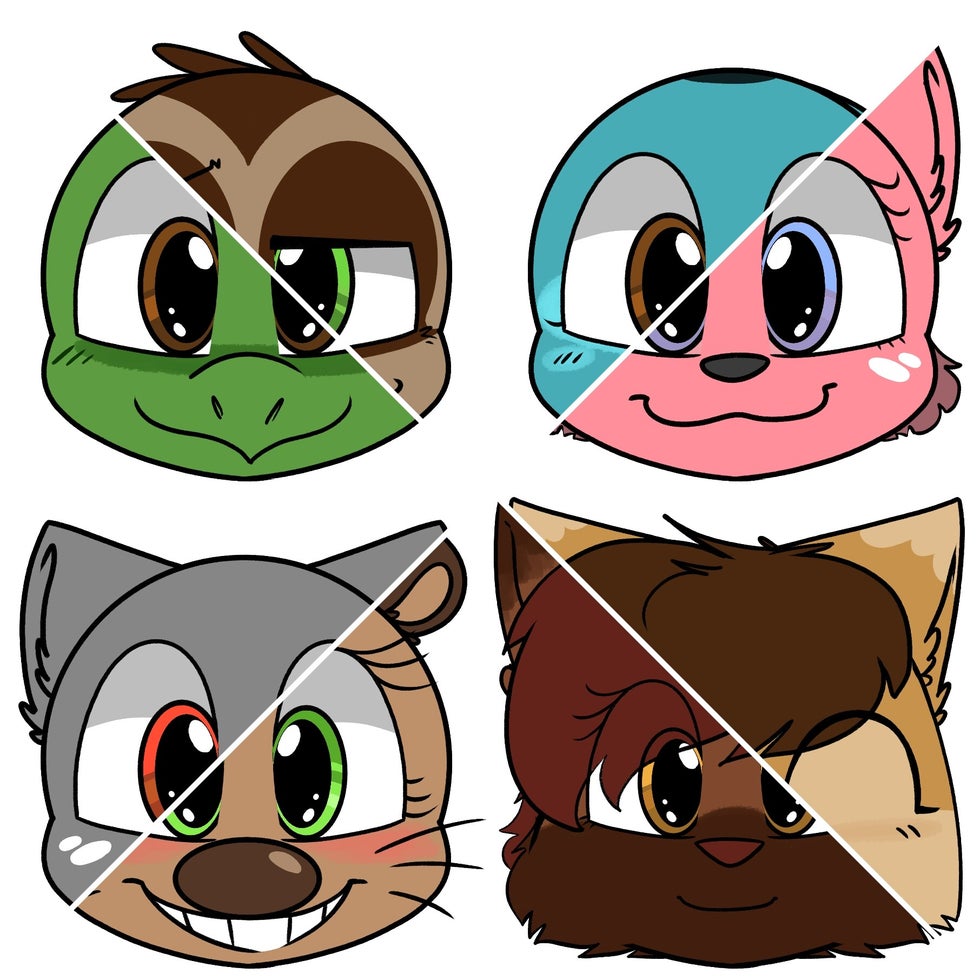
After the art assets were made, I looked into the coding, by following official GM tutorials and labelled examples, I followed them in detail and over a couple weeks I was able to make the game functional. I kept all of the notes in the code through //text to come back to when I needed it. However, I do see many ways to improve it. Currently each levels needs it's own line of code to determine objectives and score, but I feel this could be done in a better way, perhaps a global script with a line of code that says levels 1-10 are easy, 11-20 medium and 21-30 hard, with everything beyond that being randomly chosen from a table. I couldn't find a way of doing this however, but I saw others had done something similar, so I am aware it is possible.
Tracking the score per level was difficult to figure out, I ended up needing to set up a script that tracked each levels objective, score and what characters spawned. There are a maximum of 3 objectives, with a 3 star score being between 10000 and 30000 points. Each match gets you 150 score, chaining combo matches increase the multiplier of your score. The first level only has 6 characters spawn in, so it's easier to match and get combos, the second level has 7 characters, introducing the grey one, and the 3rd level has all 8 characters. If I were to continue this going forward then I would change it so that the first 5 levels had 6 characters, and every 5-10 levels you would increase the difficulty, as this was more of a shortened version to show the potential.
The following is the code for the first levels objective and character spawn rate. It has 1 objective, which is selected from the first 6 characters on the sprite sheet. The // notes explain what each part does.
switch(room)
{
// If we are setting objectives for level 1...
case rm_level_1:
// Set first objective type to 0, 1, 2 or 3.
type_0 = irandom_range(0, 3);
// Disable second objective.
type_1 = -1;
// Disable third objective.
type_2 = -1;
// Set objective amounts to values between 3 and 6.
// Unless they're disabled, in which case just set to -1.
amount_0 = irandom_range(3, 6);
amount_1 = -1;
amount_2 = -1;
break;
The score works as such:
switch(room)
{
case rm_level_1:
global.score_target = 10000;
break;
case rm_level_2:
global.score_target = 15000;
break;
case rm_level_3:
global.score_target = 20000;
break;
Another section that required understanding was how the characters dropped, how they stop, and how the game could track when it needed to drop more. This was way beyond my understanding of code, I had to download a template and tear it apart to understand. In the process I kept some of the UI elements as so much of my time was being spent on getting the code to work. I still don't think I could do this again if I tried from memory, but basically the sprites of each object are kept on the same sprite sheet, each one is given a number (starting at zero) and the game calls for a random number between 0 ad 7, it then spawns in enough to fill any blank spaces on the grid. The objects fall until they collide with the hitbox of another object which stops them. This is the super basic way of explaining how the movement works. Understanding this took a lot longer than I'd like to admit.
if(x < target_x)
{
x += 10;
y += 0;
}
if(x > target_x)
{
x += -10;
y += 0;
}
if(y < target_y)
{
draw_squish = lerp(draw_squish,0.8,0.2);
x += 0;
y += 10;
if(y >= target_y)
{
draw_squish_target = 1.25;
}
}
if(y > target_y)
{
x += 0;
y += -10;
}
if(draw_y_offset < 0)
{
draw_y_offset += 10;
}
if(image_alpha < 1)
{
image_alpha += 0.1;
}
if(!(draw_squish == draw_squish_target))
{
draw_squish = lerp(draw_squish,draw_squish_target,0.2);
if(abs(draw_squish-draw_squish_target) < 0.1)
{
draw_squish_target = 1;
}
}
The following are screenshots of current level designs, these designs are repeated with higher score requirements to finish the level. It was hard to think of level designs that would fit the grid-like design and still be functional.
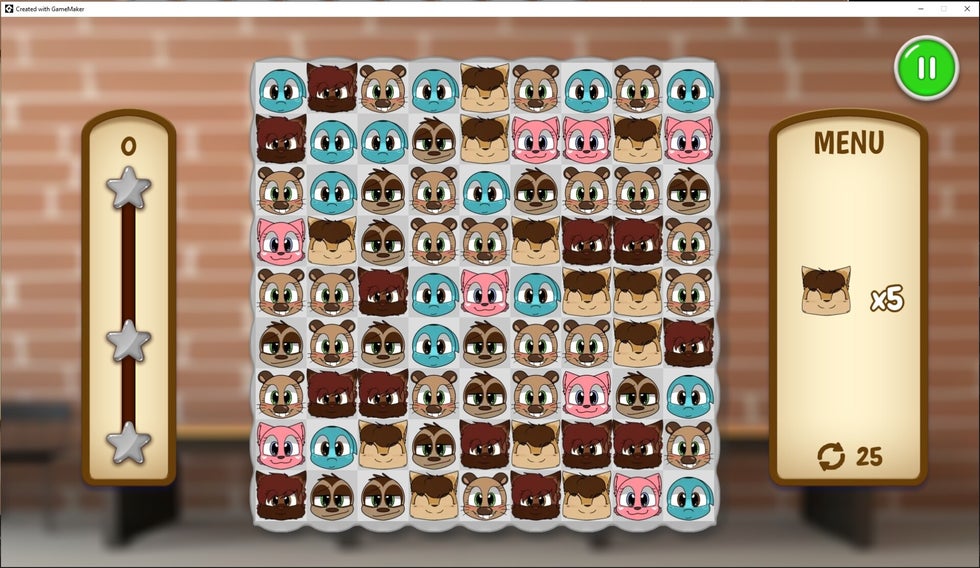
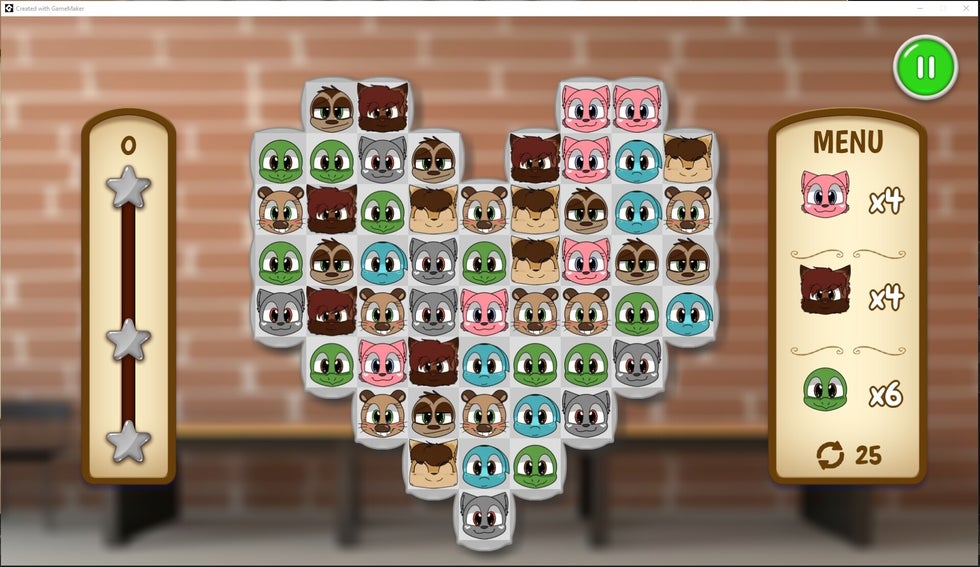
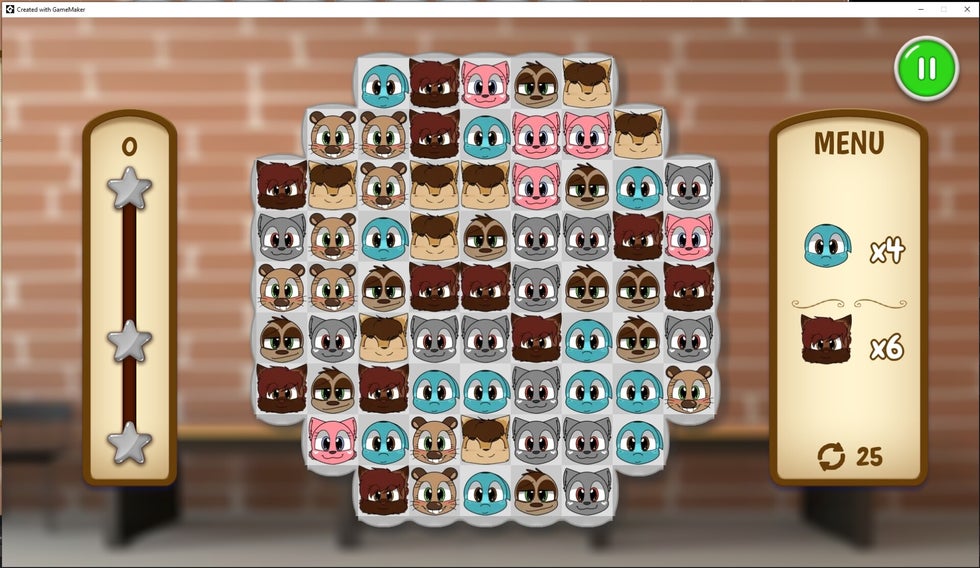
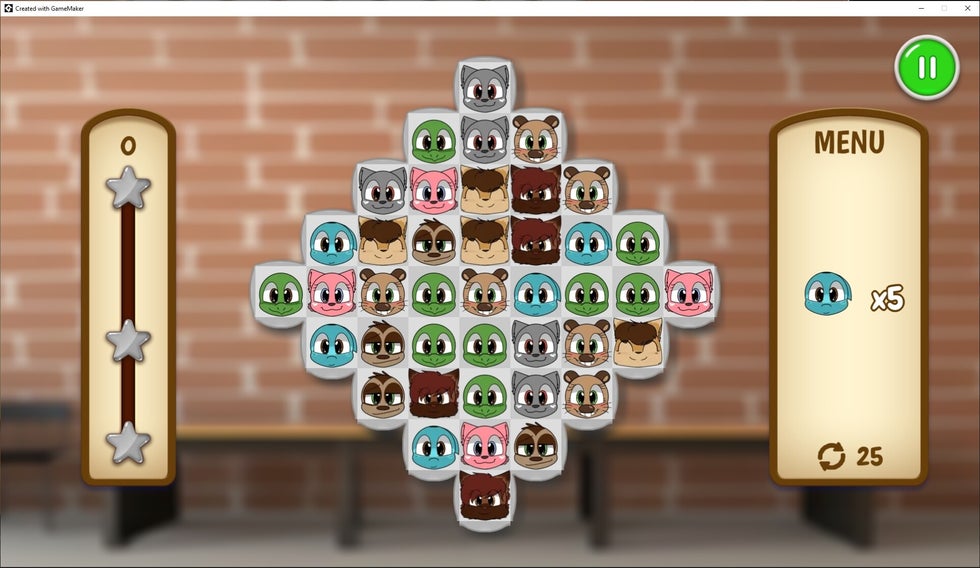
To describe how the game functions;
- There is a score counter [Left] with 3 stars that turn gold when you pass certain score thresholds.
- There is an objective [Right] that shows how many of a character you need to match to pass the level. Later levels require more characters to be matched with limited moves.
- Move counter [Bottom right] shows how many moved you have left to use before you fail the level.
- As for the interactive game element [Center], you swipe with either your fingers on a phone/tablet, or a computer mouse, to make 3 of the same character line up. Similar to other games like Candy Crush and Bejeweled.
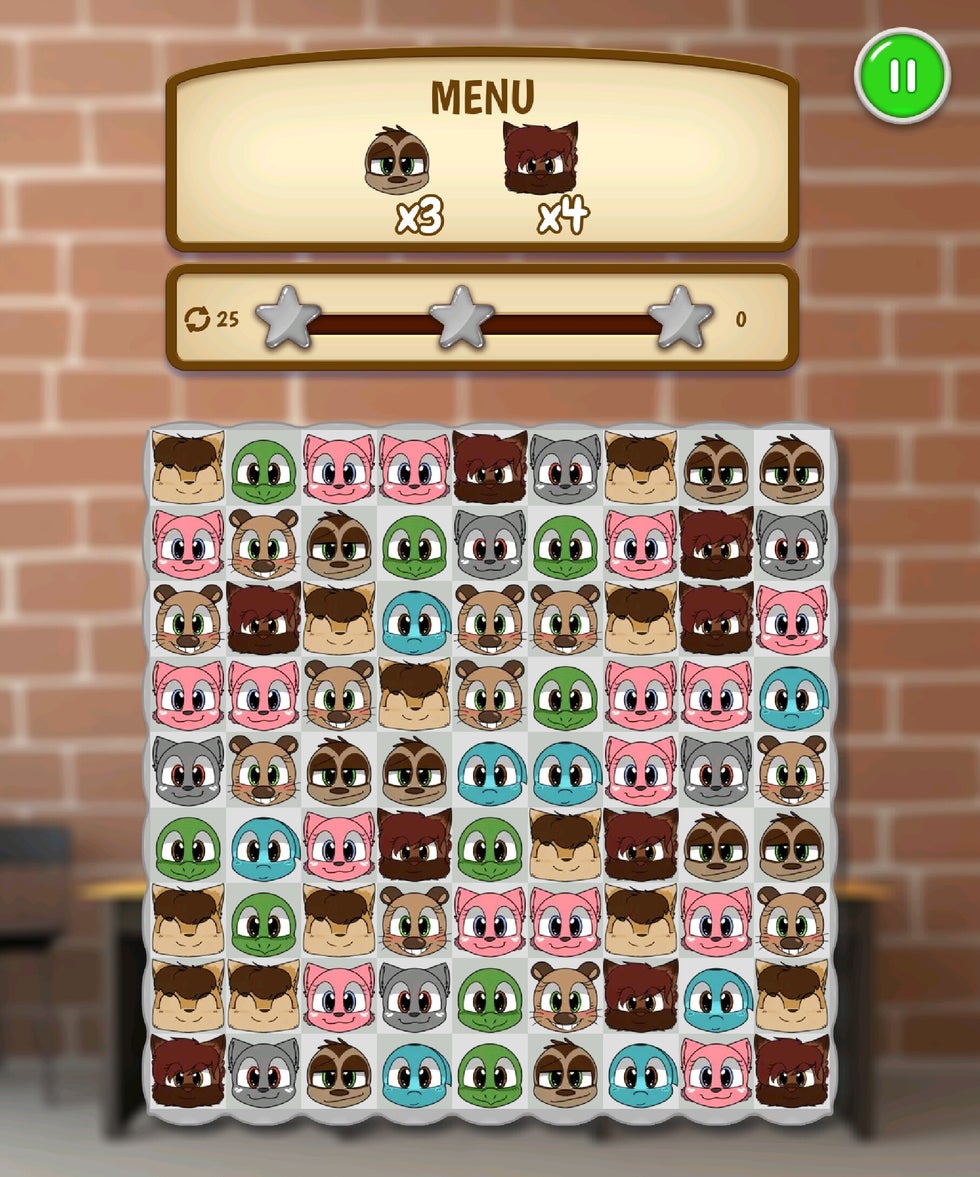
The above is a screenshot from a mobile device, showing how the game looks when played as intended with touch screen controls and on a vertical aspect ratio.
Through public testing, a common complaint was about colour, 3 characters are different shades of brown, while this may match the characters designs, I decided it was still better for players to have an enjoyable experience over having accurate character designs. Initial attempts were for the characters to appear different based on expressions, one character has their eyes half closed, another fully closed, another wide open with a toothy grin. But in practice this was not enough as these icons appear quite small on some phones. Because of this feedback, I have decided to change the colours of some of the characters so they can be easily identified at a glance.
I'm using resources on what colours to best use so that all forms of colourblindness can still enjoy the game.
https://davidmathlogic.com/colorblind/#%23D81B60-%231E88E5-%23FFC107-%23004D40
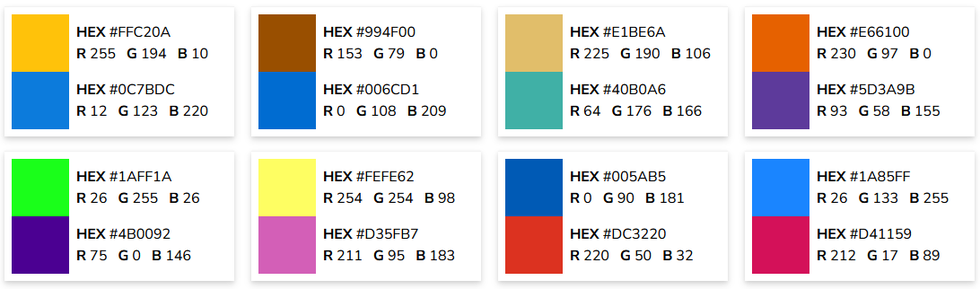
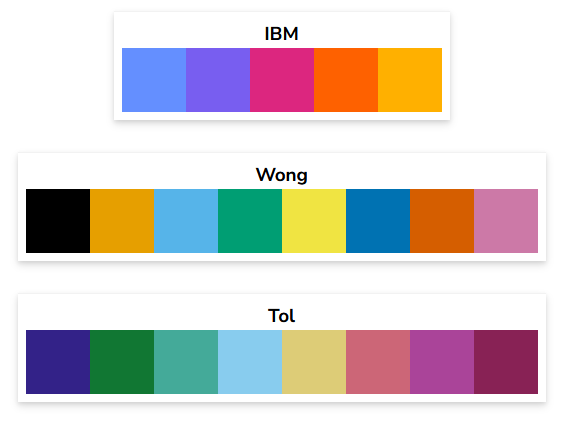
The above colour pallets are considered to be colour blind friendly, using these pallets should allow for anyone to enjoy the game.
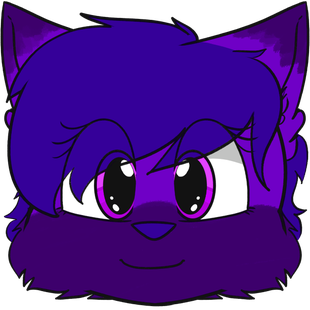
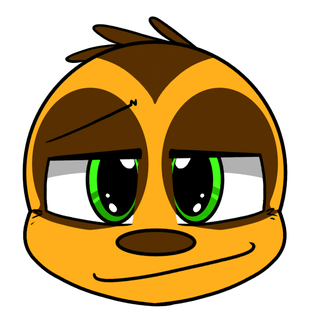
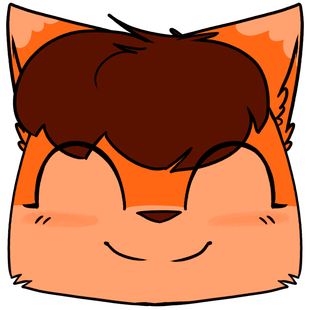
Looking at the colours above, I have decided to change 3 of the sprites for a colour blind option. It should also help anyone distinguish between characters in the game. I would like to make entirely new characters but time is quickly running out, so for now these will have to do.
You can play both versions of the game, or it's Android counterpart, by following this link: https://cccu-my.sharepoint.com/:f:/g/personal/dg355_canterbury_ac_uk/EtM7wHpadUxIk2c7DtfVApkBYnT0QORRgfmNRIW2MB90OQ?e=XT97Sq
Create Your Own Website With Webador